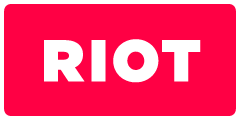
Simple and elegant component-based UI library
Custom tags • Enjoyable syntax • Elegant API • Tiny size
Why do we need a new UI library?
The frontend space is indeed crowded, but we honestly feel the solution is still “out there”. We believe Riot offers the right balance for solving the great puzzle. While React seems to do it, they have serious weak points that Riot will solve.
So — here’s why we need one:
1. Custom tags
Riot brings custom tags to all browsers.
<todo>
<!-- layout -->
<h3>{ opts.title }</h3>
<ul>
<li each={ item, i in items }>{ item }</li>
</ul>
<form onsubmit={ add }>
<input ref="input">
<button>Add #{ items.length + 1 }</button>
</form>
<!-- style -->
<style>
h3 {
font-size: 14px;
}
</style>
<!-- logic -->
<script>
this.items = []
add(e) {
e.preventDefault()
var input = this.refs.input
this.items.push(input.value)
input.value = ''
}
</script>
</todo>
A custom tag glues relevant HTML and JavaScript together forming a reusable component. Think React + Polymer but with enjoyable syntax and a small learning curve.
Human-readable
Custom tags let you build complex views with HTML. Your application might look something like this:
<body>
<h1>Acme community</h1>
<forum-header/>
<div class="content">
<forum-threads/>
<forum-sidebar/>
</div>
<forum-footer/>
<script>riot.mount('*', { api: forum_api })</script>
</body>
HTML syntax is the de facto language of the web and it’s designed for building user interfaces. The syntax is explicit, nesting is inherent to the language, and attributes offer a clean way to provide options for custom tags.
Riot tags are converted to pure JavaScript before browsers can execute them.
DOM Expressions binding
- Absolutely the smallest possible amount of DOM updates and reflows
- One way data flow: updates and unmounts are propagated downwards from parent to children
- Expressions are pre-compiled and cached for high performance
- Lifecycle events for more control
- Server-side rendering of custom tags for universal (isomorphic) applications
Close to standards
- No proprietary event system
- No need for external polyfills or additional libraries
- The rendered DOM can be freely manipulated with other tools
- No extra HTML root elements or
data-
attributes - Plays well with jQuery
Tooling friendly
- Create tags with ES6, Typescript, CoffeeScript, Jade, LiveScript or any pre-processor you want
- Integrate with NPM, CommonJS, AMD, Bower or Component
- Develop with Gulp, Grunt or Browserify plugins
2. Simple and minimalistic
Minimalism sets Riot apart from others:
1. Enjoyable syntax
One of the design goals was to introduce a powerful tag syntax with as little boilerplate as possible:
- Power shortcuts:
class={ enabled: is_enabled, hidden: hasErrors() }
- No extra brain load such as
render
,state
,constructor
- Interpolation:
Add #{ items.length + 1 }
orclass="item { selected: flag }"
- The
<script>
tag to enclose the logic is optional - Compact ES6 method syntax
2. Small learning curve
Riot has between 10 and 100 times fewer API methods than other UI libraries.
- Less to learn. Fewer books and tutorials to view
- Less proprietary stuff and more standard stuff
3. Tiny size
polymer.html – 49.38KB (gzip)
react.min.js – 33.27KB (gzip)
riot.min.js – 10.85KB (gzip)
- Fewer bugs
- Faster to parse and cheaper to download
- Embeddable. The library ought to be smaller than the application
- Less to maintain. We don’t need a big team to maintain Riot
4. Small, but complete
Riot has all the essential building blocks for modern client-side applications:
- “Reactive” views for building user interfaces
- Event library for building APIs with isolated modules
- Optional Router for taking care of URL and the back button
Riot is an “open stack”. It’s meant for developers who want to avoid framework specific idioms. The generic tools let you mix and match design patterns. Systems like Facebook Flux can be self-made.
@riotjs_ Thanks for creating a library that allows me to make a simple SPA in plain JS without needing to download 13k NPM packages. @coussej
Switched my site from #BackboneJS to #RiotJS. Other than compile/mount not blocking for DOM render, it’s great! #JavaScript @riotjs_ @richardtallent
Like all people fed up with the status quo and the development of the world, I’m voting RiotJS <3 Love you baby @plumpNation
I looked at the riot.js example, and it feels so clean, it’s scary. @paulbjensen
I liked the idea of #reactjs with #flux but I like #riotjs with #riotcontrol even better! @tscok
Played with riot.js and like it so much more than React. Minimalistic, fast and a comprehensible API. @juriansluiman
Conclusion
Riot is Web Components for everyone. Think React + Polymer but without the bloat. It’s intuitive to use and it weighs almost nothing. And it works today. No reinventing the wheel, but rather taking the good parts of what’s there and making the simplest tool possible.
We should focus on reusable components instead of templates. According to the developers of React:
“Templates separate technologies, not concerns.”
By having related layout and logic together under the same component the overall system becomes cleaner. We respect React for this important insight.